Latest Posts
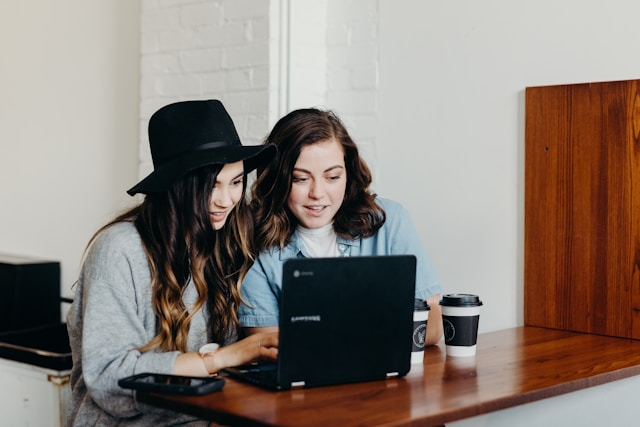
Linux User and Group Management Guide
A comprehensive guide to managing users and groups in Linux
Read Post
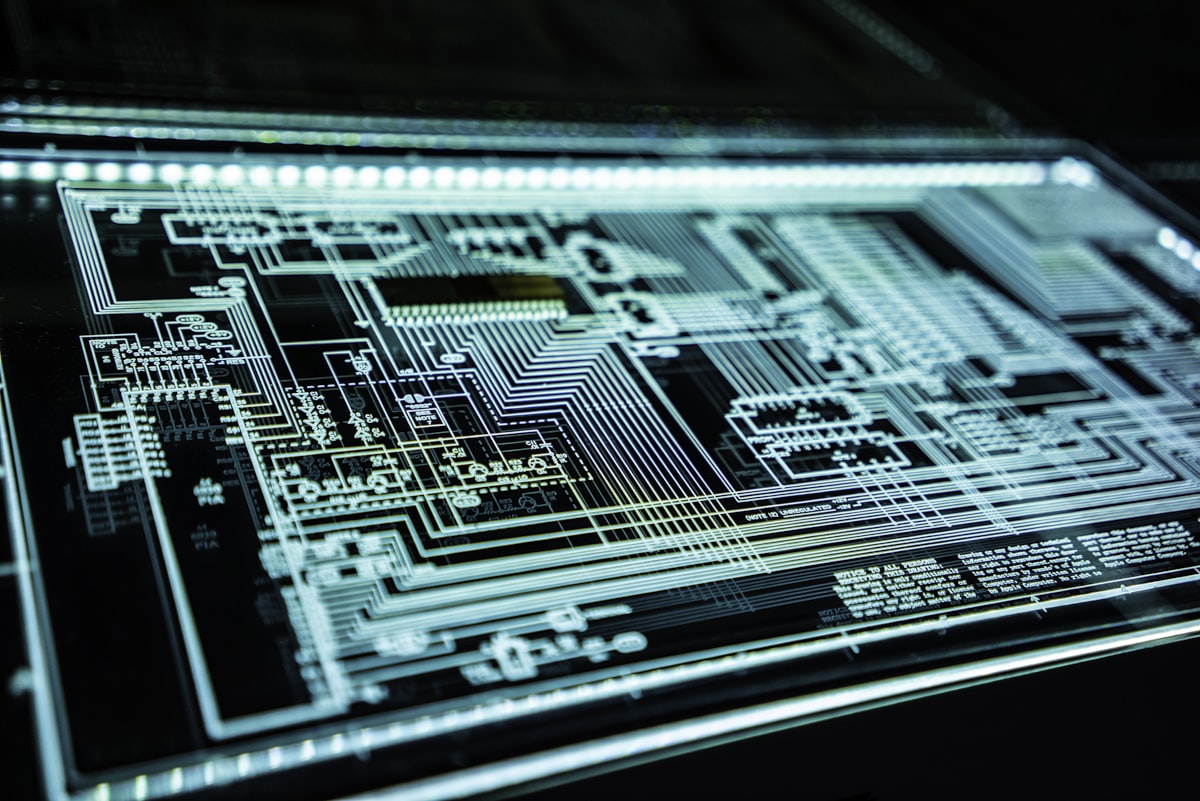
Securing Linux Filesystem
A comprehensive guide to securing Linux filesystem
Read Post
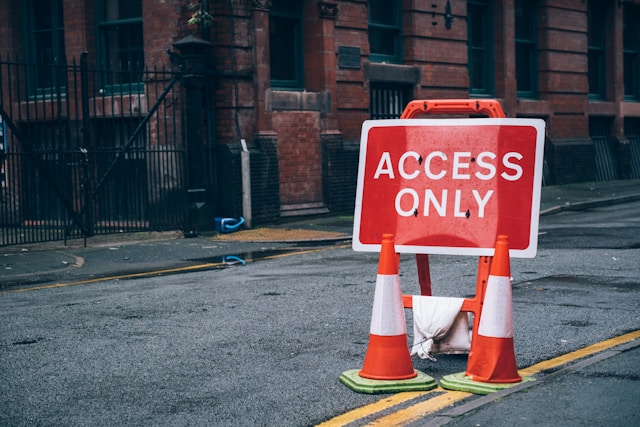
Understanding Linux File Permissions
A detailed guide to Linux file permissions and access control
Read Post
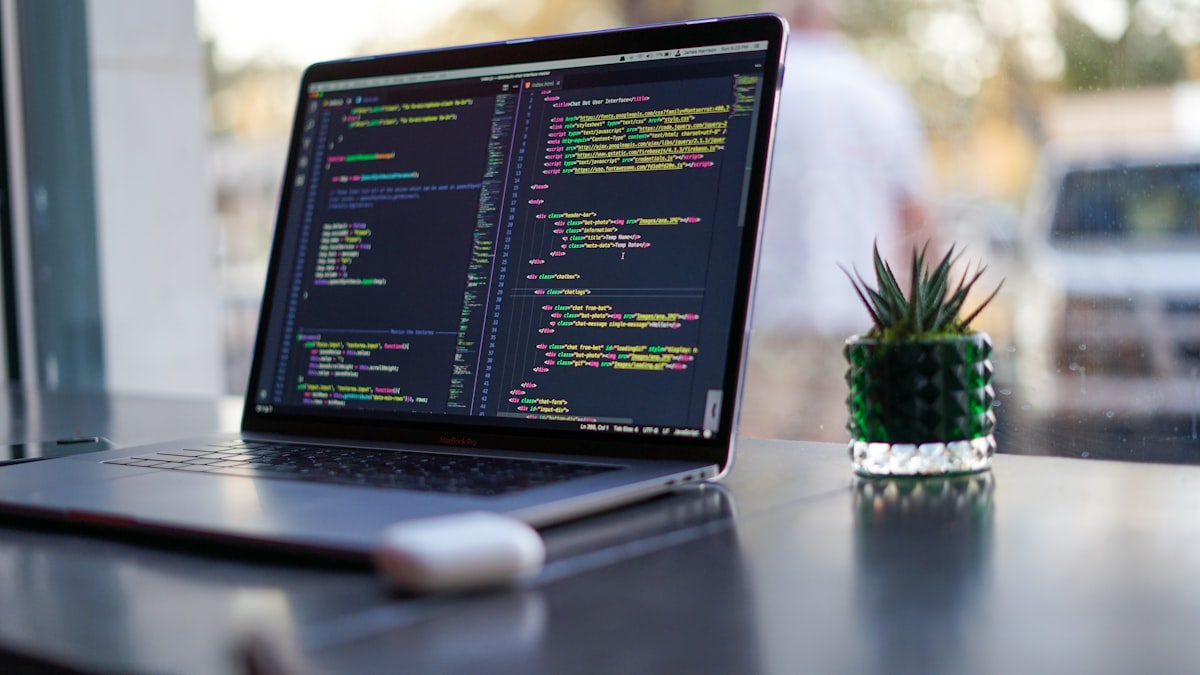
Go vs C: Modern System Programming and Performance Analysis
An in-depth comparison of Go and C for modern system programming, featuring real-world benchmarks, architectural considerations, and emerging use cases in cloud and IoT environments.
Read Post
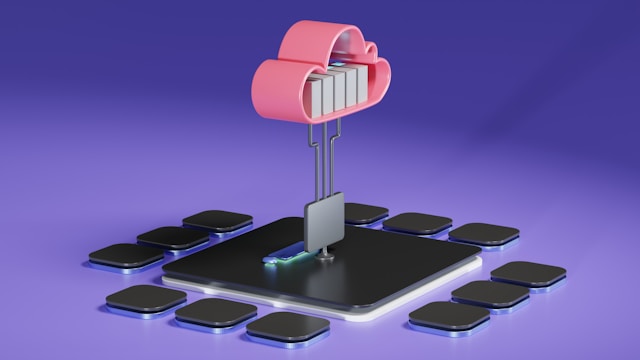
Building Cloud-Native Applications: Docker, Kubernetes, and Modern Microservices
A comprehensive guide to designing and implementing cloud-native applications using Docker, Kubernetes, and modern microservices patterns
Read Post
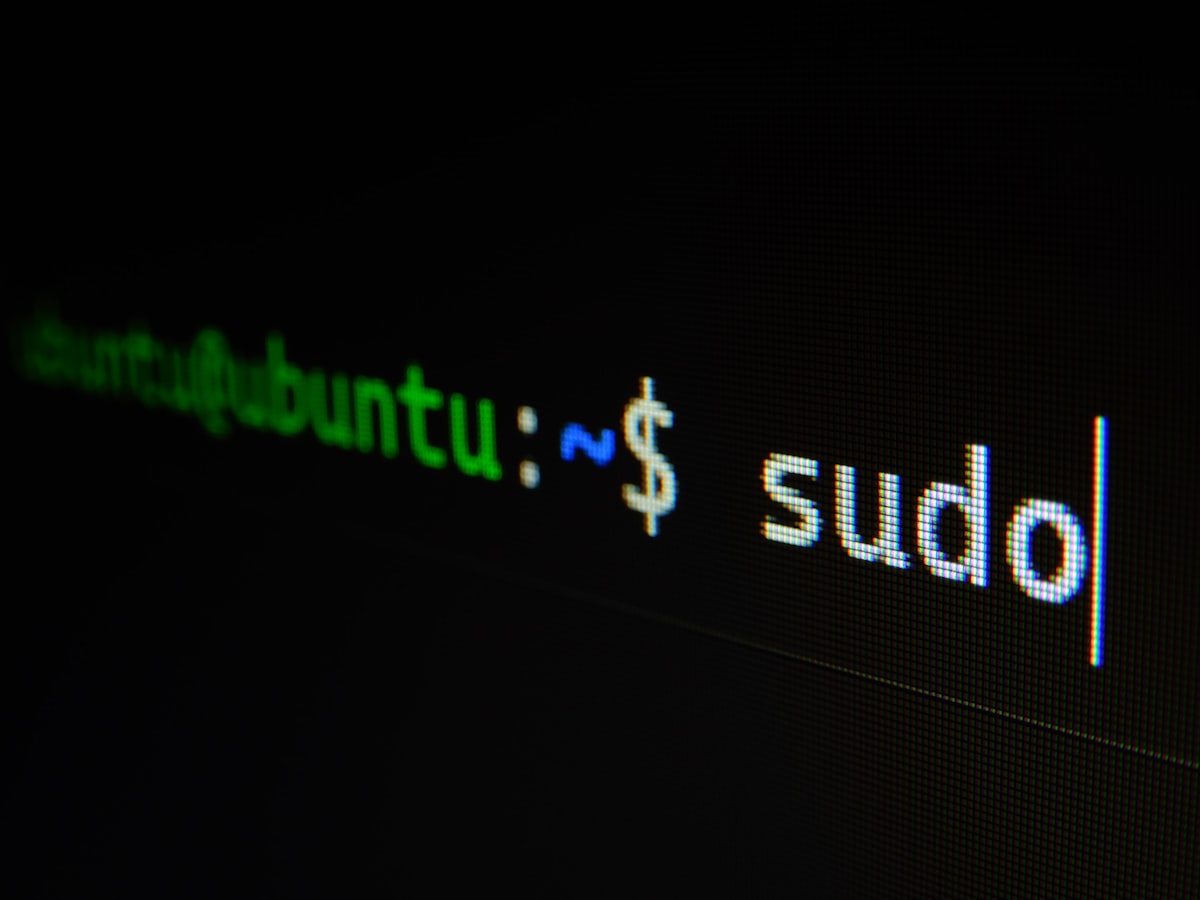
Modern Linux System Programming: A Deep Dive into 2020 Practices
Explore cutting-edge Linux system programming techniques, focusing on modern kernel features, advanced memory management, and real-world performance optimization strategies.
Read Post
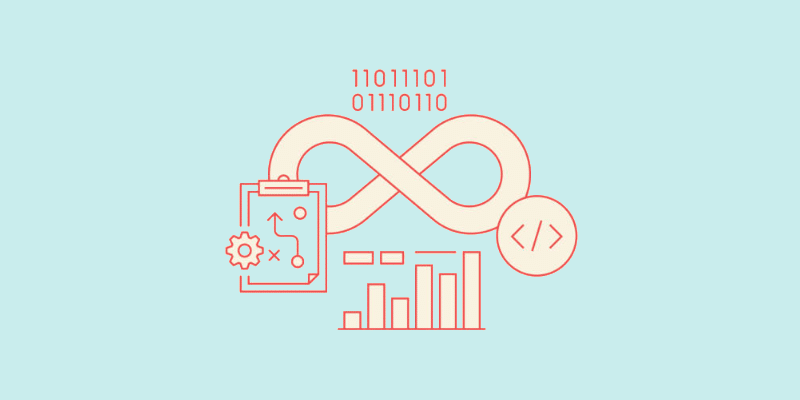
Modern DevOps Practices: Bridging Development and Operations
Explore modern DevOps practices, tools, and methodologies that are reshaping how teams deliver and maintain software applications.
Read Post